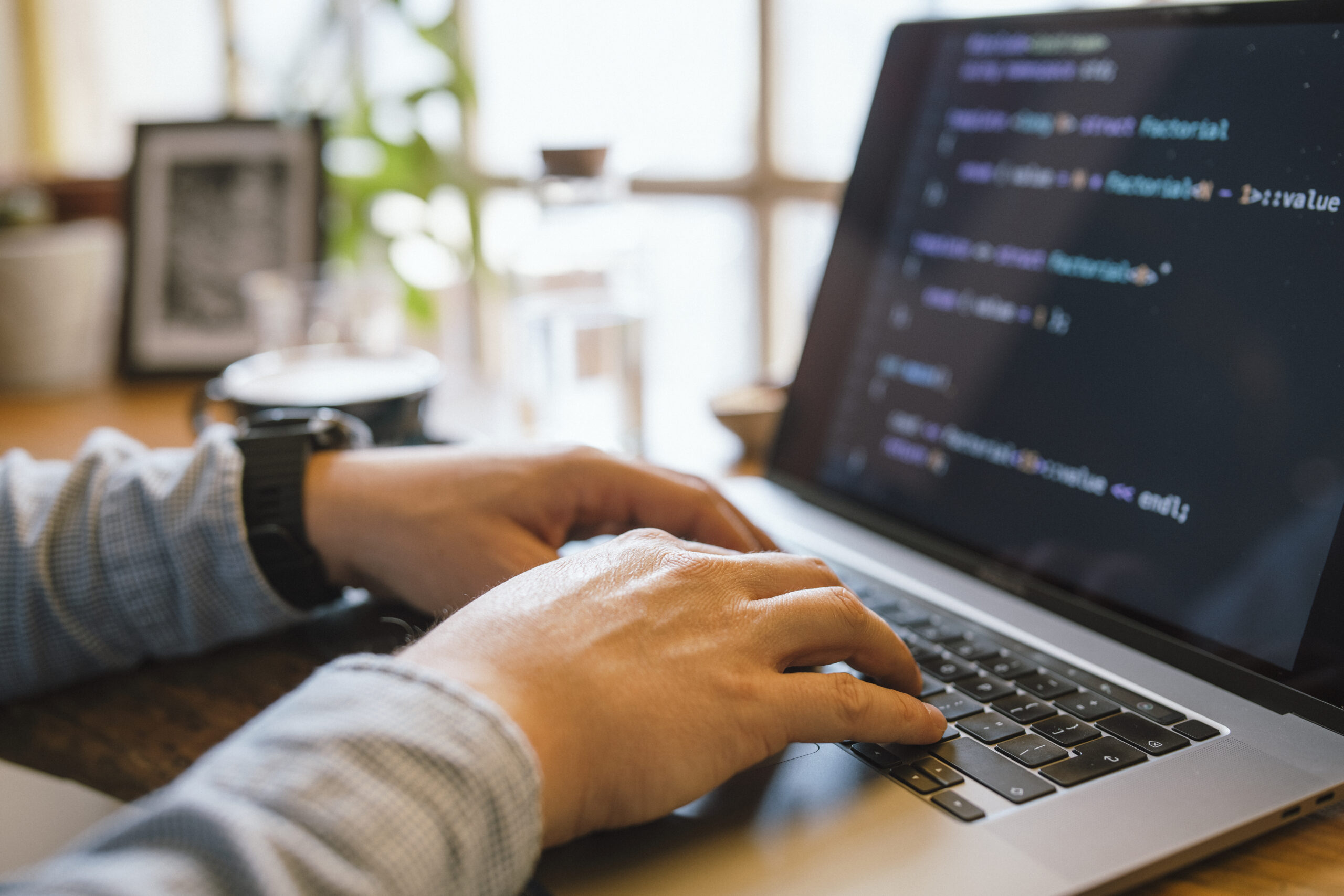
Debugging is one of the most vital — but typically missed — skills inside a developer’s toolkit. It is not almost correcting broken code; it’s about comprehension how and why matters go wrong, and Understanding to Assume methodically to unravel problems proficiently. Irrespective of whether you're a newbie or possibly a seasoned developer, sharpening your debugging techniques can help you save hours of annoyance and radically improve your productivity. Listed below are numerous techniques that will help builders level up their debugging sport by me, Gustavo Woltmann.
Master Your Tools
One of many fastest means builders can elevate their debugging capabilities is by mastering the equipment they use daily. Whilst producing code is one particular A part of development, recognizing ways to communicate with it proficiently for the duration of execution is equally significant. Present day improvement environments occur Outfitted with highly effective debugging capabilities — but lots of developers only scratch the surface of what these instruments can do.
Consider, such as, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications allow you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code about the fly. When used properly, they Enable you to observe particularly how your code behaves in the course of execution, which happens to be priceless for tracking down elusive bugs.
Browser developer instruments, such as Chrome DevTools, are indispensable for entrance-close developers. They assist you to inspect the DOM, check network requests, check out serious-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can change frustrating UI troubles into workable jobs.
For backend or system-stage developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management over working procedures and memory management. Understanding these instruments may have a steeper Understanding curve but pays off when debugging effectiveness challenges, memory leaks, or segmentation faults.
Past your IDE or debugger, become cozy with Model Regulate systems like Git to comprehend code heritage, uncover the precise minute bugs ended up released, and isolate problematic changes.
Ultimately, mastering your resources suggests likely beyond default settings and shortcuts — it’s about producing an intimate familiarity with your improvement setting to make sure that when issues come up, you’re not dropped at the hours of darkness. The greater you understand your resources, the more time it is possible to commit solving the actual trouble as an alternative to fumbling by the procedure.
Reproduce the situation
One of the more significant — and infrequently overlooked — steps in successful debugging is reproducing the trouble. Just before jumping into the code or earning guesses, developers need to produce a reliable setting or situation where the bug reliably seems. Without reproducibility, correcting a bug gets a sport of chance, generally resulting in wasted time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Question concerns like: What actions triggered The problem? Which surroundings was it in — development, staging, or output? Are there any logs, screenshots, or error messages? The more element you've got, the easier it will become to isolate the exact ailments below which the bug takes place.
When you finally’ve gathered sufficient facts, make an effort to recreate the condition in your neighborhood environment. This could signify inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem appears intermittently, take into consideration creating automatic checks that replicate the edge situations or point out transitions concerned. These checks not just enable expose the issue but in addition reduce regressions in the future.
Often, the issue could possibly be ecosystem-certain — it might take place only on certain working techniques, browsers, or underneath particular configurations. Employing tools like virtual equipment, containerization (e.g., Docker), or cross-browser testing platforms is usually instrumental in replicating this sort of bugs.
Reproducing the problem isn’t only a action — it’s a mindset. It calls for endurance, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're currently halfway to fixing it. Using a reproducible circumstance, You should utilize your debugging applications extra correctly, take a look at prospective fixes safely, and converse extra Evidently along with your group or end users. It turns an abstract complaint into a concrete obstacle — and that’s wherever builders prosper.
Read and Recognize the Mistake Messages
Mistake messages tend to be the most respected clues a developer has when a little something goes Completely wrong. Rather then observing them as frustrating interruptions, developers should really find out to take care of error messages as immediate communications within the procedure. They typically let you know just what took place, where it transpired, and at times even why it occurred — if you understand how to interpret them.
Begin by looking at the concept thoroughly As well as in entire. A lot of builders, especially when less than time pressure, look at the first line and straight away start out generating assumptions. But deeper during the mistake stack or logs might lie the legitimate root trigger. Don’t just copy and paste mistake messages into engines like google — study and have an understanding of them very first.
Crack the error down into pieces. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or operate induced it? These thoughts can guidebook your investigation and issue you toward the responsible code.
It’s also handy to know the terminology with the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Discovering to recognize these can considerably quicken your debugging course of action.
Some mistakes are obscure or generic, As well as in These situations, it’s very important to examine the context through which the mistake occurred. Check out similar log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings either. These typically precede larger concerns and supply hints about probable bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, supporting you pinpoint challenges faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Sensibly
Logging is one of the most highly effective applications inside of a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, helping you recognize what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
An excellent logging method begins with realizing what to log and at what stage. Widespread logging stages contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for thorough diagnostic data during enhancement, Facts for normal functions (like profitable commence-ups), WARN for potential issues that don’t break the applying, Mistake for real problems, and Lethal if the method can’t continue.
Stay clear of flooding your logs with too much or irrelevant facts. Excessive logging can obscure crucial messages and decelerate your process. Give attention to key situations, condition alterations, input/output values, and significant decision details as part of your code.
Format your log messages clearly and continuously. Contain context, like timestamps, ask for IDs, and function names, so it’s easier to trace troubles in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting the program. They’re Specially valuable in creation environments where stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. Which has a properly-thought-out logging strategy, you could reduce the time it will require to identify issues, achieve further visibility into your applications, and Increase the overall maintainability and dependability of your respective code.
Believe Just like a Detective
Debugging is not merely a specialized undertaking—it's a kind of investigation. To correctly determine and correct bugs, builders will have to approach the process like a detective fixing a thriller. This mindset aids break down intricate difficulties into workable parts and adhere to clues logically to uncover the root result in.
Start off by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, acquire just as much applicable information as you can with out jumping to conclusions. Use logs, test cases, and user reports to piece together a transparent picture of what’s happening.
Next, variety hypotheses. Talk to you: What may very well be resulting in this habits? Have any adjustments not too long ago been designed on the codebase? Has this concern occurred before less than very similar conditions? The aim would be to slender down possibilities and detect probable culprits.
Then, check your theories systematically. Try to recreate the condition in the controlled environment. When you suspect a particular function or component, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results direct you nearer to the truth.
Pay close awareness to tiny aspects. Bugs typically hide from the least envisioned areas—similar to a lacking semicolon, an off-by-1 mistake, or perhaps a race affliction. Be comprehensive and affected individual, resisting the urge to patch the issue without the need of completely being familiar with it. Short term fixes may perhaps cover the real issue, just for it to resurface later.
And finally, continue to keep notes on Everything you tried using and discovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term difficulties and help Other folks have an understanding of your reasoning.
By considering similar to a detective, builders can sharpen their analytical abilities, technique click here complications methodically, and turn into more practical at uncovering hidden concerns in intricate units.
Create Exams
Producing checks is among the most effective tips on how to enhance your debugging expertise and Total enhancement performance. Tests not only assist catch bugs early but in addition function a security Web that gives you self-assurance when building variations to your codebase. A nicely-tested application is easier to debug because it permits you to pinpoint specifically where and when a problem occurs.
Get started with device checks, which deal with unique functions or modules. These little, isolated exams can swiftly reveal regardless of whether a particular bit of logic is Performing as envisioned. Any time a exam fails, you straight away know where to glimpse, noticeably lessening enough time used debugging. Device assessments are Specifically beneficial for catching regression bugs—problems that reappear after Beforehand staying mounted.
Up coming, integrate integration checks and close-to-conclusion exams into your workflow. These help make sure several areas of your application do the job collectively easily. They’re particularly handy for catching bugs that take place in intricate techniques with multiple parts or providers interacting. If something breaks, your assessments can let you know which part of the pipeline unsuccessful and under what ailments.
Creating tests also forces you to definitely Believe critically regarding your code. To test a element effectively, you need to be aware of its inputs, expected outputs, and edge scenarios. This level of knowledge Normally sales opportunities to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continuously, you are able to center on repairing the bug and enjoy your take a look at pass when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, composing assessments turns debugging from the frustrating guessing activity into a structured and predictable procedure—supporting you capture much more bugs, speedier and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s simple to become immersed in the trouble—staring at your display for hrs, striving Option just after solution. But Probably the most underrated debugging equipment is just stepping away. Using breaks will help you reset your head, lower irritation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive fatigue sets in. You might begin overlooking obvious errors or misreading code that you wrote just hours earlier. Within this point out, your Mind gets considerably less productive at difficulty-solving. A brief wander, a espresso break, or perhaps switching to a different process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Specially throughout longer debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away helps you to return with renewed Electricity as well as a clearer mindset. You would possibly quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a very good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten moment split. Use that time to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, but it surely really brings about quicker and simpler debugging in the long run.
In a nutshell, using breaks will not be a sign of weak point—it’s a sensible strategy. It provides your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and relaxation is an element of resolving it.
Discover From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to mature as being a developer. No matter if it’s a syntax mistake, a logic flaw, or perhaps a deep architectural concern, each one can educate you a thing important in the event you make time to mirror and review what went wrong.
Begin by asking oneself a number of critical thoughts as soon as the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught previously with superior procedures like device screening, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or comprehension and allow you to Create more robust coding practices relocating forward.
Documenting bugs may also be an outstanding practice. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. As time passes, you’ll begin to see styles—recurring difficulties or widespread blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug using your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a brief compose-up, or a quick awareness-sharing session, supporting Other individuals avoid the exact situation boosts group performance and cultivates a more powerful Studying culture.
Additional importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers are certainly not the ones who publish ideal code, but people that constantly study from their problems.
In the end, Each and every bug you take care of adds a different layer to your ability established. So subsequent time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Conclusion
Bettering your debugging techniques requires time, follow, and persistence — although the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.